Chapter 2
Getting Started with Rust
"There are no shortcuts in evolution." — Louis Pasteur
Chapter 2 of MLVR serves as a comprehensive introduction to the Rust programming language, focusing on its unique features that make it well-suited for machine learning applications. The chapter begins with an overview of Rust’s design principles, including memory safety, concurrency without data races, and the ownership model, which are foundational to understanding Rust's advantages. Readers are guided through setting up the Rust development environment and writing their first Rust programs. The chapter then delves into key concepts such as Rust’s ownership model, error handling, and concurrency, providing both theoretical explanations and practical coding examples. Additionally, it covers how to use Rust’s package manager, Cargo, to manage projects and dependencies, and explores Rust's interoperability with other languages like C and Python. By the end of this chapter, readers will have a solid understanding of Rust’s core features and be prepared to apply them in machine learning contexts.
2.1. Introduction to Rust
Rust is a modern systems programming language that has garnered significant attention due to its innovative approach to safety, concurrency, and performance. Introduced by Mozilla Research in 2010 and first released in stable form in 2015, Rust was designed to address the limitations of existing systems languages, particularly C and C++, by providing memory safety without the need for a garbage collector and enabling concurrency without data races. Rust’s design philosophy centers around empowering developers to write safe, fast, and concurrent code without sacrificing control over system resources, making it an increasingly popular choice in both systems programming and other domains like web development, game development, and, of course, machine learning.
One of the most significant innovations that Rust brings to the table is its ownership model. At the heart of Rust’s memory safety guarantees is the ownership system, which ensures that each value in a Rust program has a single owner at any point in time. This system is complemented by Rust’s borrowing and lifetime features, which allow developers to reference data without transferring ownership, all while ensuring that references do not outlive the data they point to. These concepts are the foundation of Rust’s safety guarantees, preventing common programming errors such as null pointer dereferencing, use-after-free, and data races, which can lead to unpredictable behavior and security vulnerabilities in other languages.
Understanding Rust’s ownership model begins with the concept of ownership itself. In Rust, every piece of data is owned by a single variable, and when that variable goes out of scope, the data is automatically cleaned up. This ensures that memory is efficiently managed without the overhead of a garbage collector. Rust also allows developers to borrow references to data, either immutably or mutably, but enforces strict rules to prevent data races and ensure that borrowed references are always valid. Lifetimes in Rust are annotations that describe the scope in which a reference is valid, and they are crucial in ensuring that references do not outlive the data they reference, thereby preventing use-after-free errors.
Rust’s syntax is designed to be familiar to developers coming from other languages like C, C++, or Java, but with some unique features that support its safety and concurrency guarantees. For instance, variable bindings in Rust are immutable by default, meaning that once a value is assigned to a variable, it cannot be changed unless explicitly marked as mutable using the mut
keyword. This immutability by default encourages developers to write more predictable and thread-safe code. Another key difference in Rust’s syntax is the explicit handling of errors using the Result
and Option
types, which enforce that developers consider potential failure scenarios and handle them appropriately.
Setting up the Rust development environment is straightforward, and the first step is to install Rust itself. Rust’s toolchain includes Cargo, the official package manager and build system for Rust, which simplifies the process of managing dependencies, building projects, and running tests. To install Rust and Cargo, you can use the rustup
tool, which is the recommended way to manage Rust versions and associated tools. After installing Rust, setting up an integrated development environment (IDE) with Rust support can significantly improve the development experience. Popular editors like Visual Studio Code, IntelliJ IDEA, and Sublime Text have excellent Rust integration through plugins that provide features like syntax highlighting, code completion, and inline error checking.
To get a feel for Rust’s syntax and compiling process, let’s start with writing a simple "Hello, World!" program. This is a classic first step in learning any programming language and serves as a basic introduction to the structure of a Rust program.
fn main() {
println!("Hello, World!");
}
In this example, we define a main
function, which is the entry point of a Rust program. The println!
macro is used to print the string "Hello, World!" to the console. In Rust, macros are identified by the !
suffix and are a powerful feature that allows for metaprogramming. Unlike functions, macros can generate code at compile time, enabling more flexible and reusable code patterns.
To compile and run this program, save the code in a file with a .rs
extension, such as main.rs
. Then, open a terminal, navigate to the directory containing the file, and run the following commands:
rustc main.rs
./main
The rustc
command is Rust’s compiler, which takes the source file and compiles it into an executable. After running the executable, you should see "Hello, World!" printed in the terminal.
Alternatively, you can use Cargo to create and manage your Rust projects. Cargo simplifies the process of compiling and running Rust programs and managing dependencies. To create a new project with Cargo, run:
cargo new hello_world
cd hello_world
cargo run
Cargo creates a new directory named hello_world
, sets up the necessary files and folders, and initializes a Git repository. The src/main.rs
file contains the same "Hello, World!" code. By running cargo run
, Cargo automatically compiles the program and runs the resulting executable.
The Rust Programming Language (commonly referred to as TRPL) is the Ran’stAI book that provides an in-depth introduction to the Rust language, its syntax, features, and ecosystem. Available at [trpl.rantai.dev](https://trpl.rantai.dev), this resource is designed to guide readers from foundational Rust programming concepts to advanced topics such as memory safety, concurrency, and system-level programming. Whether you're new to programming or a seasoned developer, TRPL offers clear explanations, practical examples, and hands-on projects to help you master Rust and its unique approach to safe, efficient, and concurrent software development.
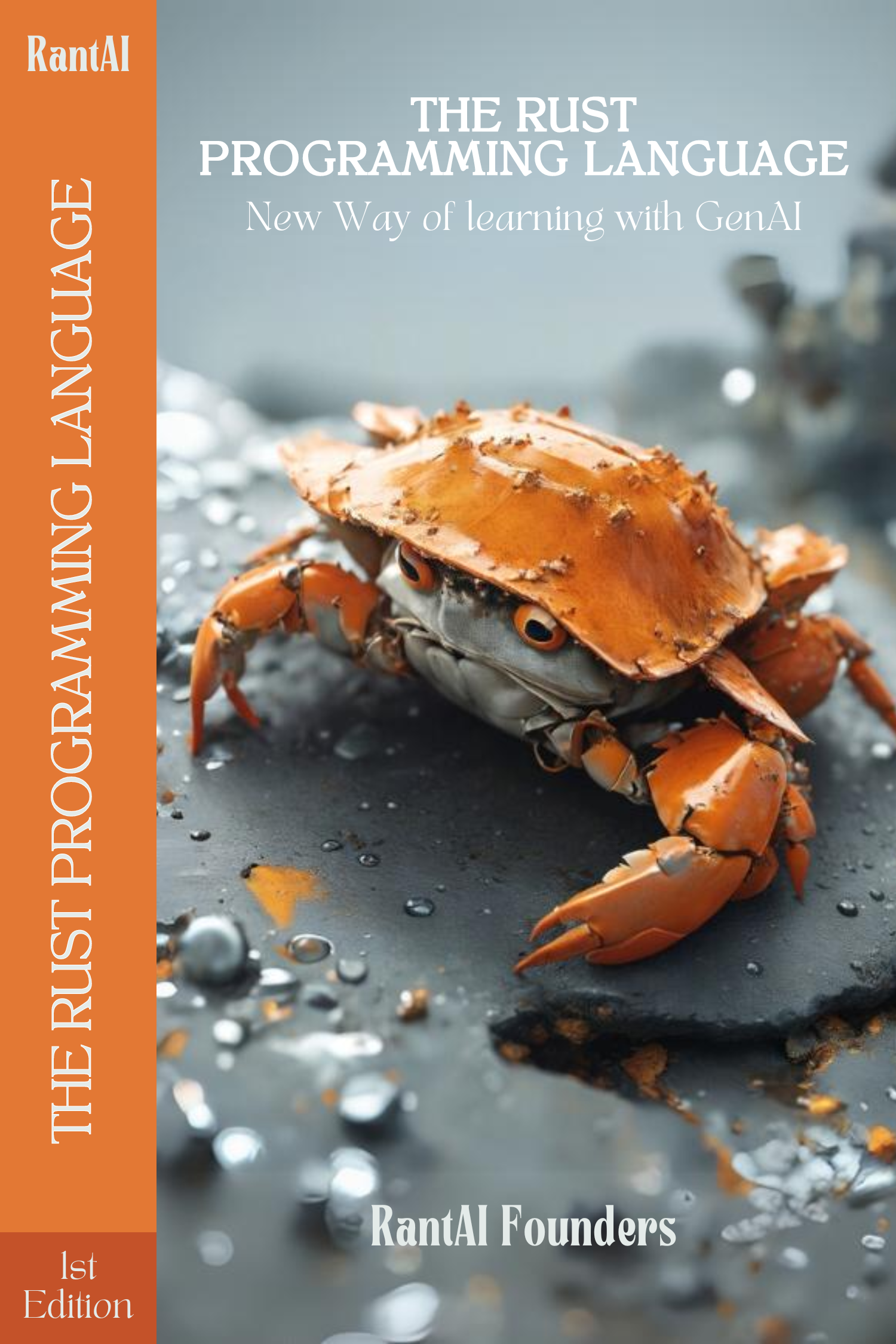
Figure 1: TRPL - The Rust Programming Language book available at https://trpl.rantai.dev.
Another book, the Modern Data Structures and Algorithms in Rust (DSAR) book, available at [dsar.rantai.dev](https://dsar.rantai.dev), provides a comprehensive guide to implementing classic and advanced data structures and algorithms using the Rust programming language. The book is designed to offer a robust exploration of foundational concepts such as stacks, queues, graphs, and dynamic programming, while also delving into practical applications like optimization and parallelism. Through detailed examples and Rust-based implementations, DSAR helps both novice and experienced machine learning developers understand how Rust's unique ownership model, type system, and concurrency features can be leveraged to write efficient and safe code for real-world applications.
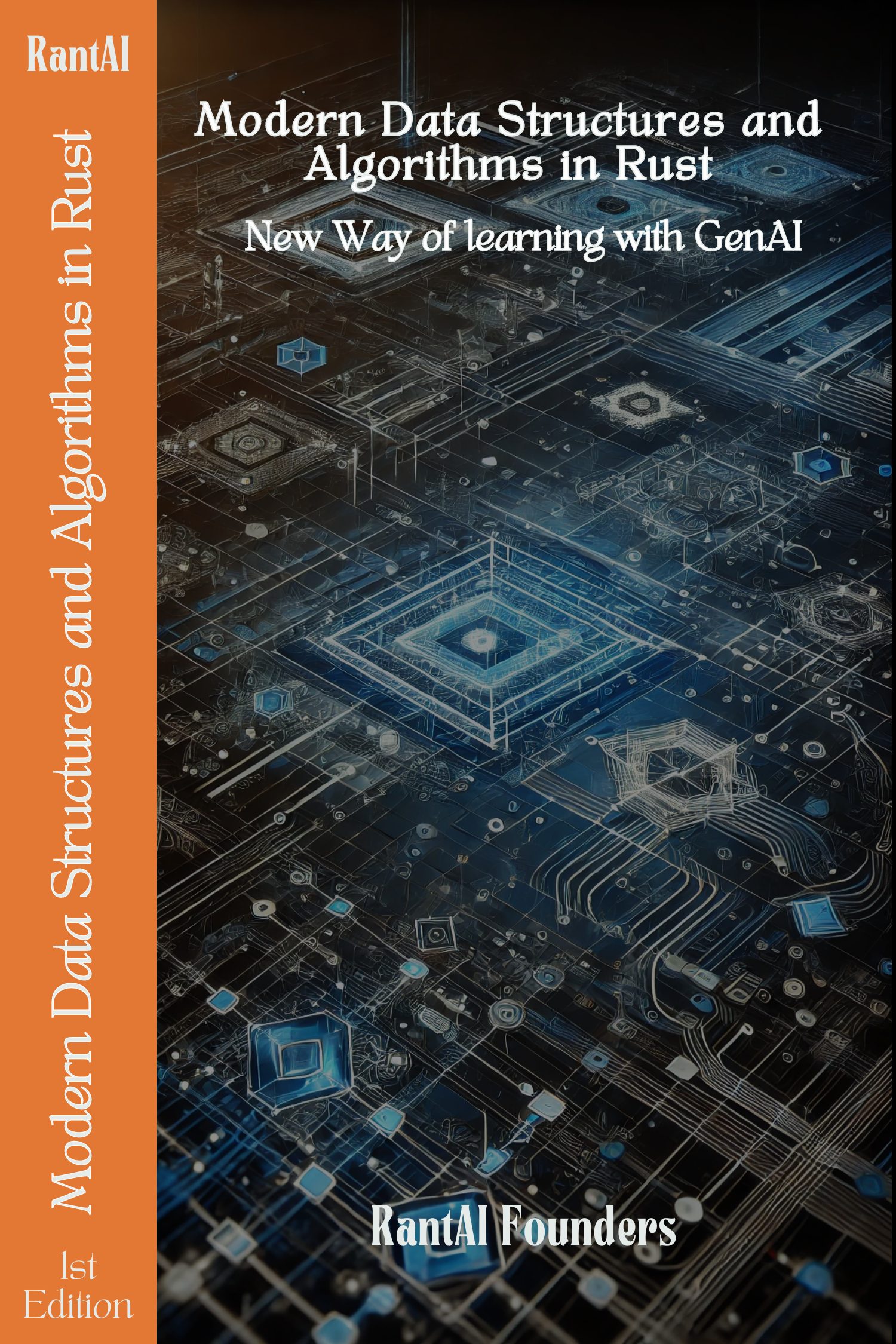
Figure 2: DSAR - Modern Data Structures and Algorithms book available at https://dsar.rantai.dev.
In conclusion, Rust is a powerful and safe systems programming language with a unique approach to memory management and concurrency. Its ownership model, combined with borrowing and lifetimes, ensures that Rust programs are free from many of the common errors that plague other systems languages. Understanding Rust’s syntax and setting up the development environment are essential first steps in getting started with Rust, and writing a simple "Hello, World!" program provides an introduction to compiling and running Rust code. As you continue to explore Rust, you will discover how its features can be leveraged to build efficient, safe, and concurrent applications, including those in the field of machine learning.
2.2. Rust’s Ownership Model
Rust’s ownership model is a cornerstone of its design, providing a unique approach to memory management that eliminates many common bugs and vulnerabilities associated with other programming languages. This model revolves around three core concepts: ownership, borrowing, and lifetimes, each of which plays a crucial role in ensuring memory safety and concurrency without a garbage collector.
Ownership in Rust is based on the principle that each value in a Rust program has a single owner at any point in time. This owner is responsible for cleaning up the value when it is no longer needed. When ownership of a value is transferred from one variable to another, Rust’s compiler enforces rules to ensure that there are no lingering references to the original value, thus preventing issues like use-after-free. This differs significantly from languages with garbage collection, where memory management is handled automatically but with some overhead and potential inefficiencies.
In Rust, when a value is assigned to another variable, ownership of that value is transferred, and the original variable is no longer valid. This concept is illustrated with the following code snippet:
fn main() {
let x = String::from("Hello, Rust!");
let y = x; // Ownership of the String is moved to y
// println!("{}", x); // This line would cause a compile-time error
println!("{}", y); // This is valid
}
In this example, the ownership of the String
object is moved from x
to y
. After the move, x
can no longer be used, as Rust enforces that x
no longer has ownership of the String
. This ensures that there is no double-free or dangling pointer issue, as y
is now the sole owner of the data.
Borrowing allows functions to temporarily use a value without taking ownership. Rust distinguishes between immutable and mutable borrowing. Immutable borrowing allows multiple parts of code to read from the same value simultaneously, while mutable borrowing allows a single part of code to modify the value but requires exclusive access. Here’s an example of immutable and mutable borrowing:
fn main() {
let s = String::from("Hello");
let s_ref1 = &s; // Immutable borrow
let s_ref2 = &s; // Another immutable borrow
println!("s_ref1: {}", s_ref1);
println!("s_ref2: {}", s_ref2);
let s_ref3 = &mut s; // Error: cannot borrow `s` as mutable, as it is not declared as mutable
s_ref3.push_str(", Rust!");
}
In this code, s
is borrowed immutably twice. Rust allows multiple immutable borrows because they do not modify the data. However, attempting to borrow s
mutably while it is already borrowed immutably results in a compile-time error. This restriction ensures that mutable borrows do not conflict with other borrows, preventing data races.
Lifetimes in Rust ensure that references are valid as long as they are needed. A lifetime is a static guarantee that a reference is valid for a certain scope. Lifetimes are particularly important in functions where references are passed as parameters or returned. The following example demonstrates lifetimes in a function:
fn longest<'a>(s1: &'a str, s2: &'a str) -> &'a str {
if s1.len() > s2.len() {
s1
} else {
s2
}
}
fn main() {
let str1 = String::from("long string");
let str2 = String::from("short");
let result = longest(&str1, &str2);
println!("The longest string is {}", result);
}
In this code, the longest
function returns a reference that is guaranteed to be valid as long as both s1
and s2
are valid. The lifetime 'a
indicates that the returned reference cannot outlive the references passed to the function. Rust’s lifetime annotations ensure that references are always valid, preventing dangling pointers and use-after-free errors.
Practical exercises to understand Rust’s ownership model can include creating programs that involve multiple ownership transfers, borrowing, and lifetime annotations. For example, writing a function that manipulates and returns various types of references can help solidify understanding of how ownership, borrowing, and lifetimes interact. Experimenting with different scenarios, such as attempting to borrow a mutable reference while an immutable reference is active, will demonstrate Rust’s safety guarantees and the compiler’s role in enforcing these rules.
2.3. Error Handling in Rust
Rust’s approach to error handling is designed to be explicit and robust, aiming to prevent many of the issues associated with error handling in other languages. Rust eschews traditional exception-based error handling, favoring a more structured approach using the Result
and Option
types. This design encourages developers to handle errors explicitly and in a controlled manner, which enhances the reliability and safety of Rust programs.
In Rust, errors are primarily represented by two types: Result
and Option
. The Result
type is used for functions that can return an error, while the Option
type is used for functions that might return a value or not. The Result
type is an enum defined as Result
, where T
is the type of the value that will be returned in the case of success, and E
is the type of the error. It has two variants: Ok(T)
, indicating success, and Err(E)
, indicating an error. The Option
type, on the other hand, is defined as Option
, with two variants: Some(T)
, indicating the presence of a value, and None
, indicating the absence of a value.
Here is a basic example of using Result
in a function that performs file I/O operations:
use std::fs::File;
use std::io::{self, Read};
fn read_file(filename: &str) -> Result<String, io::Error> {
let mut file = File::open(filename)?;
let mut contents = String::new();
file.read_to_string(&mut contents)?;
Ok(contents)
}
fn main() {
match read_file("example.txt") {
Ok(contents) => println!("File contents: {}", contents),
Err(error) => eprintln!("Error reading file: {}", error),
}
}
In this example, the read_file
function attempts to open and read a file, returning a Result
that contains either the file’s contents or an io::Error
. The ?
operator is used to propagate errors, which simplifies error handling by automatically returning an Err
if an operation fails. The main
function then uses a match
statement to handle the Result
from read_file
, distinguishing between success and error cases.
The Option
type is used when a function might not return a value. For instance, consider a function that searches for a specific value in a list:
fn find_item<'a>(items: &'a [&str], search: &str) -> Option<&'a str> {
for &item in items {
if item == search {
return Some(item);
}
}
None
}
fn main() {
let items = ["apple", "banana", "cherry"];
match find_item(&items, "banana") {
Some(item) => println!("Found: {}", item),
None => println!("Item not found"),
}
}
Here, find_item
returns an Option
containing the item if it is found, or None
if it is not. This approach clearly communicates that the search might fail and requires handling the None
case.
Rust also has the concept of panics, which occur when a program encounters an unrecoverable error. Panics are handled using the panic!
macro, which stops execution and unwinds the stack. Panics should be used sparingly, typically for situations where continuing execution does not make sense, such as bugs or critical errors. For instance:
fn divide(x: usize, y: usize) -> usize {
if y == 0 {
panic!("Attempted to divide by zero");
}
x / y
}
fn main() {
let result = divide(10, 2);
println!("Result: {}", result);
// This will cause a panic
// let result = divide(10, 0);
}
In this example, if the divisor y
is zero, the program will panic with an error message. This is appropriate when the error is something that cannot be reasonably handled at runtime.
Rust’s philosophy behind error handling is to encourage developers to handle errors explicitly. By using Result
and Option
, Rust makes error handling a part of the type system, ensuring that errors are addressed in a way that is visible and clear. This design avoids the pitfalls of unchecked returns and exception handling, where errors can be overlooked or mishandled. By requiring explicit handling of errors, Rust promotes writing more reliable and robust code.
To practice implementing these concepts, consider creating functions that perform various operations and return Result
or Option
types. Implement error handling in these functions using match
statements and the ?
operator. Additionally, explore using panic!
for scenarios where errors are catastrophic and cannot be recovered from. Exercises such as reading from files, handling user input, or performing network operations will provide practical experience with Rust’s error handling mechanisms.
2.4. Rust’s Concurrency Model
Rust’s concurrency model is a key feature that allows developers to write safe and efficient concurrent code. At its core, Rust’s approach to concurrency is characterized by "fearless concurrency," a concept that leverages the language's ownership and type system to ensure that concurrent programming is safe and free from common pitfalls such as data races and race conditions.
Rust provides concurrency through multiple mechanisms, including threads, asynchronous programming with async
/await
, and message passing using channels. These features work together to offer a robust framework for developing concurrent applications.
The fundamental concept of Rust’s concurrency model is that it extends the ownership and borrowing rules to concurrent contexts. This means that Rust's guarantees of memory safety and absence of data races apply not just to single-threaded code but also to multi-threaded and asynchronous code. For instance, Rust's type system ensures that mutable data is only accessed by one thread at a time, thus preventing data races.
Creating and managing threads in Rust is straightforward thanks to the std::thread
module. Threads in Rust are spawned using the thread::spawn
function, which takes a closure and runs it on a new thread. The following example demonstrates how to create multiple threads that perform concurrent tasks:
use std::thread;
fn main() {
let handle1 = thread::spawn(|| {
for i in 1..5 {
println!("Thread 1 - count: {}", i);
thread::sleep(std::time::Duration::from_millis(100));
}
});
let handle2 = thread::spawn(|| {
for i in 1..5 {
println!("Thread 2 - count: {}", i);
thread::sleep(std::time::Duration::from_millis(100));
}
});
handle1.join().unwrap();
handle2.join().unwrap();
}
In this example, two threads are spawned, each printing a sequence of numbers. The join
method ensures that the main thread waits for both spawned threads to complete before exiting. This simple approach allows you to execute tasks concurrently and ensures that threads are properly synchronized.
Rust’s async
/await
syntax facilitates asynchronous programming by allowing functions to run concurrently without the complexity of manual thread management. The async
keyword marks a function as asynchronous, while the await
keyword is used to pause execution until a future value is available. Here is an example of how to use async
/await
in Rust:
// Append Cargo.toml
[dependencies]
tokio = { version = "1.40.0", features = ["full"] }
use tokio;
#[tokio::main]
async fn main() {
let task1 = async {
for i in 1..5 {
println!("Async task 1 - count: {}", i);
tokio::time::sleep(tokio::time::Duration::from_millis(100)).await;
}
};
let task2 = async {
for i in 1..5 {
println!("Async task 2 - count: {}", i);
tokio::time::sleep(tokio::time::Duration::from_millis(100)).await;
}
};
let (result1, result2) = tokio::join!(task1, task2);
}
In this code, the tokio
runtime is used to execute asynchronous tasks. The try_join!
macro is used to run both tasks concurrently and wait for their completion. Asynchronous programming with async
/await
helps in writing non-blocking code that is more readable and maintainable compared to traditional threading.
Message passing is another concurrency mechanism in Rust, facilitated by channels. Channels provide a way for threads to communicate by sending messages between them. The std::sync::mpsc
module provides the basic functionality for channels. Here is an example of using channels to send and receive messages between threads:
use std::sync::mpsc;
use std::thread;
fn main() {
let (tx, rx) = mpsc::channel();
let producer = thread::spawn(move || {
for i in 1..5 {
tx.send(i).unwrap();
thread::sleep(std::time::Duration::from_millis(100));
}
});
let consumer = thread::spawn(move || {
while let Ok(message) = rx.recv() {
println!("Received: {}", message);
}
});
producer.join().unwrap();
consumer.join().unwrap();
}
In this example, a producer thread sends integers to a channel, while a consumer thread receives and prints these integers. The mpsc::channel
function creates a new channel, and send
and recv
methods are used to pass messages between threads.
Practical exercises in Rust’s concurrency model might involve parallelizing simple algorithms, such as computing the sum of an array or processing items in parallel. For instance, you could write a program that divides a large array into chunks, processes each chunk in parallel, and then combines the results.
By leveraging Rust’s concurrency features, such as threads, async
/await
, and channels, you can write safe and efficient concurrent code. Rust’s ownership and borrowing rules ensure that concurrent programming remains safe, preventing issues like data races and ensuring that data is accessed in a controlled manner. Understanding and applying these concurrency concepts is crucial for developing high-performance and reliable Rust applications.
2.5. Using Rust’s Cargo and Crates
Cargo is an integral part of the Rust programming ecosystem, serving as both a package manager and a build system. It simplifies the management of Rust projects, automates various tasks related to building, testing, and maintaining code, and plays a crucial role in leveraging Rust’s rich ecosystem of libraries and tools.
Cargo provides a streamlined way to create and manage Rust projects. When you start a new Rust project, Cargo initializes a project structure with a Cargo.toml
file, which is the heart of the project's configuration. This file includes metadata about the project, such as its name, version, and dependencies. Here’s how you can set up a new Rust project with Cargo:
cargo new my_project
cd my_project
The cargo new
command creates a new directory named my_project
with a default project structure, including a src
directory with a main.rs
file and a Cargo.toml
file. The Cargo.toml
file automatically includes a [dependencies]
section where you can add external libraries, known as crates, that your project depends on.
Crates are Rust’s way of distributing libraries and tools. The Rust ecosystem relies heavily on crates, which are available through crates.io, the Rust package registry. Using Cargo, you can add dependencies to your project by specifying them in the Cargo.toml
file. For instance, if you want to use the serde
crate for serialization and deserialization, you would modify Cargo.toml
like this:
[dependencies]
serde = "1.0"
Once you’ve updated Cargo.toml
, Cargo will automatically download the specified crate and its dependencies when you build your project. To build the project, you simply run:
cargo build
Cargo handles compiling your code, managing dependencies, and generating the final executable. If you need to run tests, Cargo provides a straightforward command to do so:
cargo test
This command runs all the tests defined in your project and provides detailed output on their results. Testing is a crucial part of maintaining code quality, and Cargo’s integration with testing frameworks makes it easy to ensure that your code behaves as expected.
Cargo also supports benchmarking, which is useful for measuring the performance of your code. To add a benchmark, you create a benches
directory in your project and place benchmark code in it. You can then run benchmarks with:
cargo bench
This command executes the benchmarks and provides performance metrics, allowing you to identify and optimize performance bottlenecks.
Another powerful feature of Cargo is its ability to publish crates to crates.io. If you’ve developed a crate that you want to share with the Rust community, you can publish it using Cargo. First, you need to create an account on crates.io and add your API key to your Cargo configuration. Then, you can publish your crate with:
cargo publish
Publishing your crate makes it available for others to use and contributes to the growing ecosystem of Rust libraries and tools.
Here’s a simple example of creating a crate and publishing it. Suppose you want to create a crate that provides basic arithmetic operations. You would start by creating a new library crate:
cargo new my_math_lib --lib
This command generates a library project with a src/lib.rs
file. You can add functions to lib.rs
, such as:
pub fn add(x: i32, y: i32) -> i32 {
x + y
}
pub fn subtract(x: i32, y: i32) -> i32 {
x - y
}
After writing your crate, you update Cargo.toml
with metadata and ensure that your crate is well-documented and tested. Once you are ready to publish, you run cargo publish
and your crate will be available on crates.io.
2.6. Integrating Rust with Other Languages
Rust's design not only emphasizes memory safety and concurrency but also provides robust mechanisms for interoperability with other programming languages. This capability is crucial for leveraging existing libraries and systems or for integrating Rust into larger, multi-language projects. This section explores how Rust interacts with other languages, particularly C and Python, through its Foreign Function Interface (FFI).
The Foreign Function Interface (FFI) is a set of features in Rust that allows it to call functions and use data structures defined in other programming languages. This interoperability is essential for integrating Rust with C, a language with a rich set of existing libraries and a long history of use in systems programming, as well as Python, known for its extensive ecosystem and ease of use. Rust's FFI capabilities enable developers to write performance-critical components in Rust while maintaining the ability to interact with codebases written in other languages.
When considering integration with other languages, the primary motivation often revolves around performance and leveraging existing libraries. Rust excels in performance due to its low-level control and zero-cost abstractions, making it an ideal choice for components where performance is critical. By integrating Rust with C or Python, developers can optimize performance-intensive parts of their applications while still benefiting from the high-level abstractions and extensive libraries provided by these languages.
To illustrate integrating Rust with C, consider a scenario where you want to create a Rust library that exposes a function to C code. First, you'll need to define a Rust library and use the #[no_mangle]
attribute to prevent Rust from changing the names of the functions when compiling, which ensures that C code can link to them. Here is a simple example of a Rust library that exposes a function to C:
// src/lib.rs
#[no_mangle]
pub extern "C" fn add(a: i32, b: i32) -> i32 {
a + b
}
To compile this library, use Cargo to build it as a C-compatible dynamic library:
cargo build --release
This will generate a shared library file (e.g., libmylib.so
on Linux, mylib.dll
on Windows) that you can link against from C code. Here’s a C program that uses this Rust library:
// main.c
#include <stdio.h>
extern int add(int a, int b);
int main() {
int result = add(5, 7);
printf("Result: %d\n", result);
return 0;
}
To compile and link the C code with the Rust library, you can use a command like:
gcc -o main main.c -L./target/release -lmylib
For Python integration, Rust can interface with Python through tools like PyO3, which provides a way to write Python extensions in Rust. PyO3 allows Rust functions to be called from Python and vice versa, facilitating the use of Rust’s performance benefits in Python applications. Here’s an example of exposing a Rust function to Python using PyO3:
First, add pyo3
and maturin
to your Cargo.toml
:
[dependencies]
pyo3 = { version = "0.18", features = ["extension-module"] }
[lib]
crate-type = ["cdylib"]
Then, define a Rust function and set up the PyO3 module:
// src/lib.rs
use pyo3::prelude::*;
#[pyfunction]
fn add(a: usize, b: usize) -> usize {
a + b
}
#[pymodule]
fn mymodule(py: Python, m: &PyModule) -> PyResult<()> {
m.add_function(wrap_pyfunction!(add, m)?)?;
Ok(())
}
Build the Python module with maturin
:
maturin develop
You can then import and use the Rust function in Python:
import mymodule
result = mymodule.add(5, 7)
print("Result:", result)
Integrating Rust with other languages brings several practical considerations and limitations. When using Rust with C, issues such as memory management and data representation need careful handling to ensure compatibility. For Python integration, understanding Python’s Global Interpreter Lock (GIL) and managing the interplay between Rust’s concurrency model and Python’s execution model is crucial.
2.7. Conclusion
By the end of Chapter 2, you will have developed a deep understanding of Rust’s core features, empowering you to write safe, efficient, and concurrent programs. This foundation is crucial as you begin to apply Rust to complex machine learning problems, where the language’s unique strengths will become invaluable.
2.7.1. Further Learning with GenAI
Each prompt encourages detailed exploration, ensuring a robust comprehension of Rust's features and their practical use cases.
Compare Rust's ownership model to memory management techniques in other programming languages, such as C++'s manual memory management and Java's garbage collection. How does Rust ensure memory safety without a garbage collector, and what are the implications for performance and concurrency?
Explain in detail how Rust's borrowing and lifetimes work, particularly in the context of complex data structures like trees and graphs. How do these concepts prevent common memory errors such as dangling references, and what are the challenges of implementing lifetimes in deeply nested structures?
Discuss the philosophy behind Rust's approach to error handling with the Result and Option types. How does this approach differ from exception-based error handling in languages like Java or Python, and what are the trade-offs in terms of code readability, maintainability, and safety?
Analyze the benefits and limitations of Rust's pattern matching and the ? operator for error propagation. In what scenarios might these features lead to more robust code, and are there situations where they might obscure error handling or lead to subtle bugs?
Provide an in-depth explanation of Rust's concurrency model, focusing on the concepts of ownership and data races. How does Rust's compile-time guarantees compare to runtime checks in languages like Python's GIL or Java's synchronized methods? Discuss specific scenarios where Rust's model excels.
Explore the implementation of multi-threading in Rust, including the use of thread pools, channels, and locks. How do Rust’s ownership and borrowing rules interact with concurrency primitives to prevent data races, and what are the challenges of balancing safety with performance in highly concurrent systems?
Discuss the async/await paradigm in Rust. How does it compare to traditional threading models and other asynchronous programming paradigms in languages like JavaScript or Python? Provide examples of both IO-bound and CPU-bound tasks to illustrate the strengths and limitations of Rust's async/await.
Explain the concept of message passing in Rust using channels. How do channels ensure thread safety and avoid deadlocks in concurrent applications? Compare this approach to shared-memory concurrency models and discuss scenarios where message passing might be preferable.
Dive into the role of Cargo in the Rust ecosystem. How does Cargo facilitate project management, dependency resolution, and building processes compared to tools like Maven for Java or npm for JavaScript? Discuss the advantages of Cargo's approach to versioning and reproducibility in large-scale projects.
Analyze the Rust crate ecosystem, focusing on the most important crates for machine learning and data processing. How do these crates extend Rust’s functionality, and what are the key considerations when choosing crates for a machine learning project? Discuss how to evaluate crate quality, maintainability, and community support.
Explore the process of creating and managing a Rust project using Cargo. Discuss best practices for organizing code into modules, handling dependencies, and structuring tests and benchmarks. How does Cargo’s approach compare to other build systems in terms of flexibility, performance, and ease of use?
Explain how to create and publish a Rust crate. What are the steps involved in preparing a crate for publication, including writing documentation, adding tests, and ensuring compatibility across Rust versions? Discuss common challenges in maintaining an open-source crate and how to address them.
Discuss Rust’s interoperability with C using the Foreign Function Interface (FFI). How does Rust ensure safety when interacting with C code, and what are the best practices for minimizing risks such as undefined behavior or memory leaks? Provide examples of integrating a C library into a Rust project.
Examine the use of PyO3 to integrate Rust with Python. How does PyO3 facilitate calling Rust functions from Python, and what are the performance implications of using Rust for CPU-bound tasks within a Python-based machine learning pipeline? Discuss scenarios where this integration would be most beneficial.
Analyze the benefits and challenges of using Rust to optimize performance-critical components in a Python-based machine learning system. Provide detailed examples of scenarios where Rust can significantly improve performance, such as in computationally intensive algorithms or real-time data processing.
Compare and contrast the trade-offs involved in integrating Rust with other programming languages, such as C and Python. Discuss the complexities of managing memory, error handling, and data interoperability across language boundaries, and provide best practices for ensuring a seamless integration.
Provide an in-depth analysis of Rust’s memory safety guarantees, focusing on how ownership, borrowing, and lifetimes work together to prevent common memory errors. Discuss specific scenarios where these features have a significant impact on program correctness, especially in complex, multithreaded applications.
Explain the concept and importance of lifetimes in Rust. How do lifetimes interact with Rust’s ownership model to prevent dangling references and ensure memory safety? Provide examples of complex lifetime annotations in scenarios such as multi-threaded environments and deeply nested data structures.
Discuss the role of the Rust community in the development and evolution of the Rust ecosystem. How do community-driven projects, open-source contributions, and community feedback influence the development of Rust, particularly in areas like machine learning and systems programming?
Explore the future of Rust in the machine learning domain. What are the current limitations of Rust for machine learning, and how might these be addressed in future developments? Discuss how Rust’s features could be leveraged to meet the growing demands of data science and AI, and what role Rust might play in the future of machine learning technology.
As you explore these topics, you’ll gain insights that are critical for mastering Rust and applying it effectively in complex machine learning scenarios. Each prompt is an opportunity to push the boundaries of your knowledge, to think critically about the language’s design, and to explore how these concepts translate into real-world applications. Embrace this journey of discovery and let it fuel your passion for learning and innovation in the world of machine learning via Rust.
2.7.2. Hands On Practices
These exercises are designed to be rigorous and challenging, pushing your skills and understanding of Rust to the next level. By completing these tasks, you'll gain deep insights into the practical applications of Rust in machine learning, along with the experience of tackling complex, real-world problems.
Task:
Design and implement a complex, custom data structure in Rust, such as a self-balancing binary search tree (e.g., AVL tree) or a graph with weighted edges and cycles. The implementation must strictly adhere to Rust’s ownership, borrowing, and lifetime rules to ensure memory safety and efficiency.
Challenges:
Implement advanced functionality such as rebalancing the tree or finding the shortest path in the graph using Dijkstra's algorithm. Carefully manage the lifetimes of nodes or graph edges, especially in cases where nodes need to borrow data from one another. Ensure that all operations, such as insertion, deletion, and traversal, respect Rust's borrowing rules and avoid common pitfalls like dangling pointers or data races.
Analysis:
After implementing the data structure, analyze its performance in terms of time complexity and memory usage. Reflect on how Rust’s ownership model influenced your design choices, particularly in ensuring thread safety and preventing memory leaks or race conditions.
Task:
Develop a high-throughput Rust application, such as a web server or a parallel file processing system, that requires robust error handling and recovery mechanisms. The application should handle various types of errors gracefully, ensuring that it can recover from failures without crashing or corrupting data.
Challenges:
Implement complex error handling using Rust’s Result and Option types, including chaining and custom error types. Develop a strategy for recovering from errors, such as retry logic, logging, or fallback operations. Incorporate error handling into both synchronous and asynchronous operations, using Rust’s async/await syntax where appropriate.
Analysis:
Stress-test the application under high load conditions, inducing errors intentionally (e.g., by simulating network failures, corrupted files, or resource exhaustion). Evaluate how well the application handles these scenarios and whether the error recovery mechanisms you implemented maintain the application's stability and performance.
Task:
Build a sophisticated data processing pipeline in Rust that leverages both parallel and asynchronous processing. The pipeline should handle a large volume of data, such as processing a real-time data stream (e.g., financial market data) or a massive dataset stored in a distributed system.
Challenges:
Implement a multi-stage pipeline where each stage processes data concurrently, using Rust’s async/await for IO-bound tasks and threads for CPU-bound tasks. Manage complex dependencies between stages, ensuring that data is correctly passed and synchronized without introducing deadlocks or data races. Use channels or other concurrency primitives to coordinate between threads, and implement backpressure mechanisms to handle varying processing speeds between stages.
Analysis:
Evaluate the performance of the pipeline, particularly in terms of throughput, latency, and resource utilization. Discuss the challenges you faced in managing concurrency in Rust and how the language’s features (e.g., ownership model, lifetimes) helped or complicated the implementation. Consider the trade-offs between parallelism and concurrency, and reflect on how Rust’s tools for managing these aspects influenced the design of your pipeline.
Task:
Design and implement a hybrid machine learning system that integrates Rust, Python, and C to leverage the strengths of each language. The system should involve a Rust component that performs performance-critical computations (e.g., matrix operations, optimization routines), a Python interface for ease of use and flexibility, and a C library for specialized functionality (e.g., hardware acceleration).
Challenges:
Implement the Rust component and expose its functionality to Python using PyO3. Integrate a C library with Rust using Foreign Function Interface (FFI), ensuring that the Rust code safely interacts with the C code and handles any potential errors or undefined behavior. Develop a Python wrapper that seamlessly integrates the Rust and C components, allowing end-users to interact with the system as if it were a pure Python library.
Analysis:
Conduct performance benchmarks to compare the Rust and C components against equivalent Python implementations. Evaluate the complexity and challenges of integrating Rust with both Python and C, particularly in managing memory safety, error handling, and data interoperability. Reflect on the benefits and drawbacks of using a multi-language approach in a machine learning system, and discuss the potential scalability and maintainability issues that may arise.
Task:
Develop a high-performance Rust crate designed for a specific machine learning task, such as implementing a custom loss function for deep learning models or an optimized algorithm for feature selection. The crate should include complex dependencies, both within the Rust ecosystem and potentially external C/C++ libraries.
Challenges:
Design the crate to be both performant and easy to use, with a focus on efficient memory management and minimal runtime overhead. Write comprehensive unit tests and integration tests to ensure the crate's correctness and robustness. Implement benchmarks to measure the crate's performance against existing solutions, identifying areas where Rust's unique features provide a competitive advantage.
Analysis:
After developing the crate, evaluate the trade-offs between performance, usability, and complexity. Reflect on the process of managing dependencies, particularly when integrating external libraries, and discuss how Cargo facilitated or complicated this process. Publish the crate to crates.io and analyze the feedback from the Rust community, considering how the crate could be improved or extended based on real-world usage.
The challenges presented here will not only enhance your technical abilities but also prepare you for the demands of advanced machine learning systems where efficiency, safety, and scalability are paramount. Remember, the journey to mastery is not easy, but the rewards are well worth the effort. Embrace the challenges, learn from the difficulties, and let your curiosity drive you to achieve excellence in Rust and machine learning.